How-To: Deploy AWS resources
Categories:
This how-to guide will show you:
- How to model an AWS S3 resource in Bicep
- How to use a sample application to interact with AWS S3 bucket
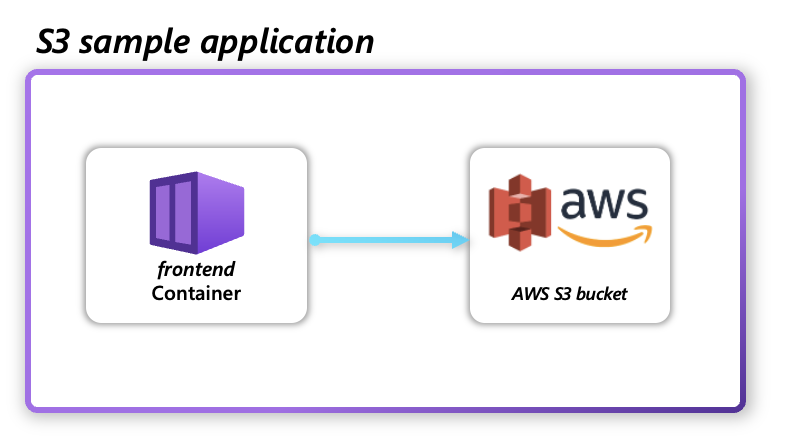
Prerequisites
- Make sure you have an AWS account and an IAM user
- Create an IAM AWS access key and copy the AWS Access Key ID and the AWS Secret Access Key to a secure location for use later. If you have already created an Access Key pair, you can use that instead.
- AWS CLI
- Configure your CLI with
aws configure
, specifying your configuration values
- Configure your CLI with
- eksctl CLI
- kubectl CLI
- rad CLI
- Radius Bicep VSCode extension
Step 1: Create an EKS Cluster
Create an EKS cluster by using the eksctl
CLI.
eksctl create cluster --name <my-cluster> --region=<my-region>
Step 2: Create a Radius Environment with the AWS cloud provider
Create a Radius Environment where you will deploy your application.
Run rad init --full
to initialize a new environment into your current kubectl context:
rad init --full
Follow the prompts to install the control plane services, create an environment resource, and create a local workspace. You will be asked for:
- Namespace - When an application is deployed, this is the namespace where your containers and other Kubernetes resources will be run. By default, this will be in the
default
namespace.💡 About namespaces
When you initialize a Radius Kubernetes environment, Radius installs the control plane resources within theradius-system
namespace in your cluster, separate from your applications. The namespace specified in this step will be used for your application deployments. - Add AWS provider - An AWS cloud provider allows you to deploy and manage AWS resources as part of your application. Follow the how-to guides to configure the AWS provider with the preferred identity.
- Environment name - The name of the environment to create. You can specify any name with lowercase letters, such as
myawsenv
.
Step 3: Create a Bicep file to model AWS Simple Storage Service (S3)
Create a new file called app.bicep
and add the following bicep code to model an AWS S3 Bucket:
import aws as aws
@description('The name of your S3 bucket.The AWS S3 Bucket name must follow the naming conventions described at https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html')
param bucket string ='mys3bucket-${uniqueString(resourceGroup().id)}'
resource s3 'AWS.S3/Bucket@default' = {
alias: bucket
properties: {
BucketName: bucket
}
}
Radius uses the AWS Cloud Control API to interact with AWS resources. This means that you can model your AWS resources in Bicep and Radius will be able to deploy and manage them. You can find the list of supported AWS resources in the AWS resource library.
Step 4: Add a Radius container to interact with the AWS S3 Bucket
Open the app.bicep
and append the following Radius resources:
@description('The environment ID of your Radius Application. Set automatically by the rad CLI.')
param environment string
resource app 'Applications.Core/applications@2023-10-01-preview' = {
name: 's3app'
properties: {
environment: environment
}
}
@description('IAM Access Key ID')
@secure()
param aws_access_key_id string
@description('IAM Access Key Secret')
@secure()
param aws_secret_access_key string
@description('Region where the S3 bucket is created. This will be the same region that you input when adding AWS cloud provider to an environment in Radius.')
param aws_region string = 'us-west-2'
// get a radius container which uses the s3 bucket
resource frontend 'Applications.Core/containers@2023-10-01-preview' = {
name: 'frontend'
properties: {
application: app.id
container: {
env: {
BUCKET_NAME: s3.properties.BucketName
AWS_ACCESS_KEY_ID: aws_access_key_id
AWS_SECRET_ACCESS_KEY: aws_secret_access_key
AWS_DEFAULT_REGION: aws_region
}
image: 'ghcr.io/radius-project/samples/aws:latest'
}
}
}
Your final app.bicep
file should look like this
import aws as aws
import radius as radius
@description('The name of your S3 bucket.The AWS S3 Bucket name must follow the [following naming conventions](https://docs.aws.amazon.com/AmazonS3/latest/userguide/bucketnamingrules.html).')
param bucket string ='mys3bucket'
resource s3 'AWS.S3/Bucket@default' = {
alias: bucket
properties: {
BucketName: bucket
}
}
@description('The environment ID of your Radius Application. Set automatically by the rad CLI.')
param environment string
resource app 'Applications.Core/applications@2023-10-01-preview' = {
name: 's3app'
properties: {
environment: environment
}
}
@description('IAM Access Key ID')
@secure()
param aws_access_key_id string
@description('IAM Access Key Secret')
@secure()
param aws_secret_access_key string
@description('Region where the S3 bucket is created. This will be the same region that you input when adding AWS cloudprovider to an environment in Radius.')
param aws_region string = 'us-west-2'
// get a radius container which uses the s3 bucket
resource frontend 'Applications.Core/containers@2023-10-01-preview' = {
name: 'frontend'
properties: {
application: app.id
container: {
env: {
BUCKET_NAME: s3.properties.BucketName
AWS_ACCESS_KEY_ID: aws_access_key_id
AWS_SECRET_ACCESS_KEY: aws_secret_access_key
AWS_DEFAULT_REGION: aws_region
}
image: 'ghcr.io/radius-project/samples/aws:latest'
}
}
}
This creates a container that will be deployed to your Kubernetes cluster. This container will interact with the AWS S3 Bucket you created in the previous step.
Step 5: Deploy the application
-
Deploy your application to your environment:
rad deploy ./app.bicep -p aws_access_key_id=<AWS_ACCESS_KEY_ID> -p aws_secret_access_key=<AWS_SECRET_ACCESS_KEY>
Replace
<AWS_ACCESS_KEY_ID>
and<AWS_SECRET_ACCESS_KEY>
with the values obtained from the previous step.Warning
It is always recommended to have separate IAM credentials for your container to communicate with S3 or any other data store. Radius is currently working on supporting direct connections to AWS resources so that your container can automatically communicate with the data store securely without having to manage separate credentials for data plane operations -
Port-forward the container to your machine with
rad resource expose
:rad resource expose containers frontend -a s3app --port 5234
-
Visit localhost:5234 in your browser. This is a swagger doc for the sample application. You can use this to interact with the AWS S3 Bucket you created. For example, you can try to upload a file to the bucket via the
/upload
endpoint.
Step 6: Cleanup
-
When you’re done with testing, you can use the rad CLI to delete an environment to delete all Radius resources running on the EKS Cluster.
-
Cleanup AWS Resources - AWS resources are not deleted when deleting a Radius Environment, so make sure to delete all resources created in this reference app to prevent additional charges. You can delete these resources in the AWS Console or via the AWS CLI. Instructions to delete an AWS S3 Bucket are available here.
Troubleshooting
If you hit errors while deploying the application, please follow the steps below to troubleshoot:
-
Check if the AWS credentials are valid. Login to the AWS console and check if the IAM access key and secret access key are valid and not expired.
-
Look at the control plane logs to see if there are any errors. You can use the following command to view the logs:
rad debug-logs
Inspect the UCP logs to see if there are any errors
If you have issues with the sample application, where the container doesn’t connect with the S3 bucket, please follow the steps below to troubleshoot:
-
Use the below command to inspect logs from container:
rad resource logs containers frontend -a s3app
Also make sure to open an Issue if you encounter a generic Internal server error
message or an error message that is not self-serviceable, so we can address the root error not being forwarded to the user.
Further Reading
Feedback
Was this page helpful?
Glad to hear it! Please feel free to star our repo and join our Discord server to stay up to date with the project.
Sorry to hear that. If you would like to also contribute a suggestion visit and tell us how we can improve.