How To: Service to service networking
Categories:
This guide will show you how two services can communicate with each other. In this example, we will have a frontend container service that communicates with a backend container service.
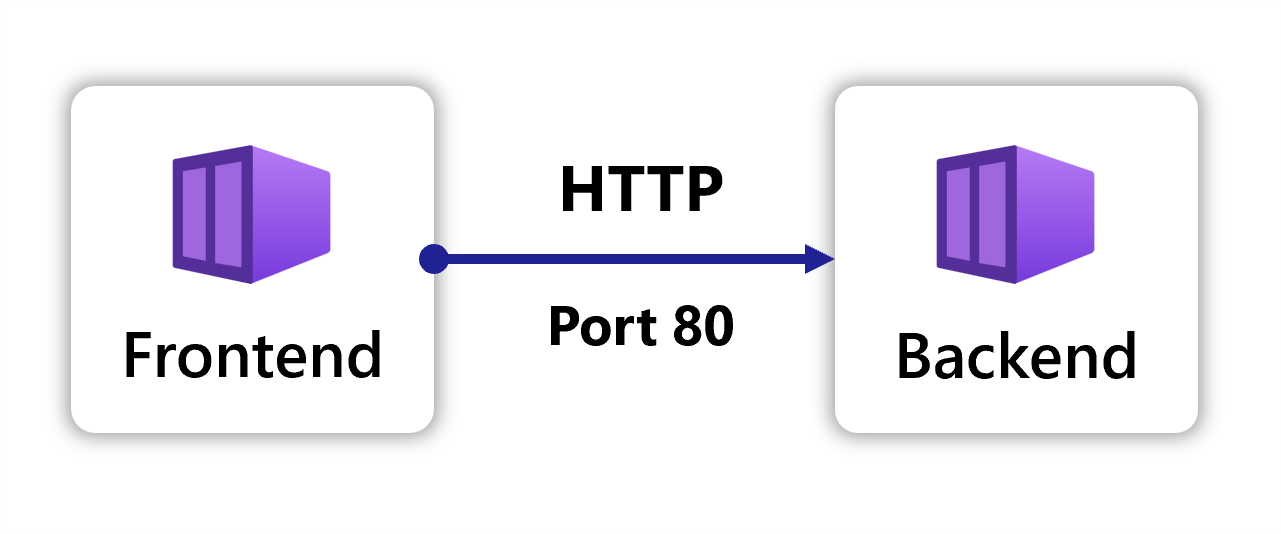
Prerequisites
Step 1: Define the services
First, define the containers in a file named app.bicep
. We will define two services: frontend
and backend
:
|
|
Note the frontend container doesn’t yet have a connection to the backend container. We will add that in the next step.
Step 2: Add a connection
With the services defined, we can now add the connection between them. Add a connection to frontend
:
|
|
Step 3: Deploy the application
Deploy the application using the rad deploy
command:
rad run app.bicep -a networking-demo
You should see the application deploy successfully and the log stream start:
Building app.bicep...
Deploying template 'app.bicep' for application 'networking-demo' and environment 'default' from workspace 'default'...
Deployment In Progress...
Completed backend Applications.Core/containers
Completed frontend Applications.Core/containers
Deployment Complete
Resources:
backend Applications.Core/containers
frontend Applications.Core/containers
Starting log stream...
Step 4: Test the connection
Visit http://localhost:3000 in your browser. You should see a connection to the backend container, along with the environment variables that have automatically been set on the frontend container:
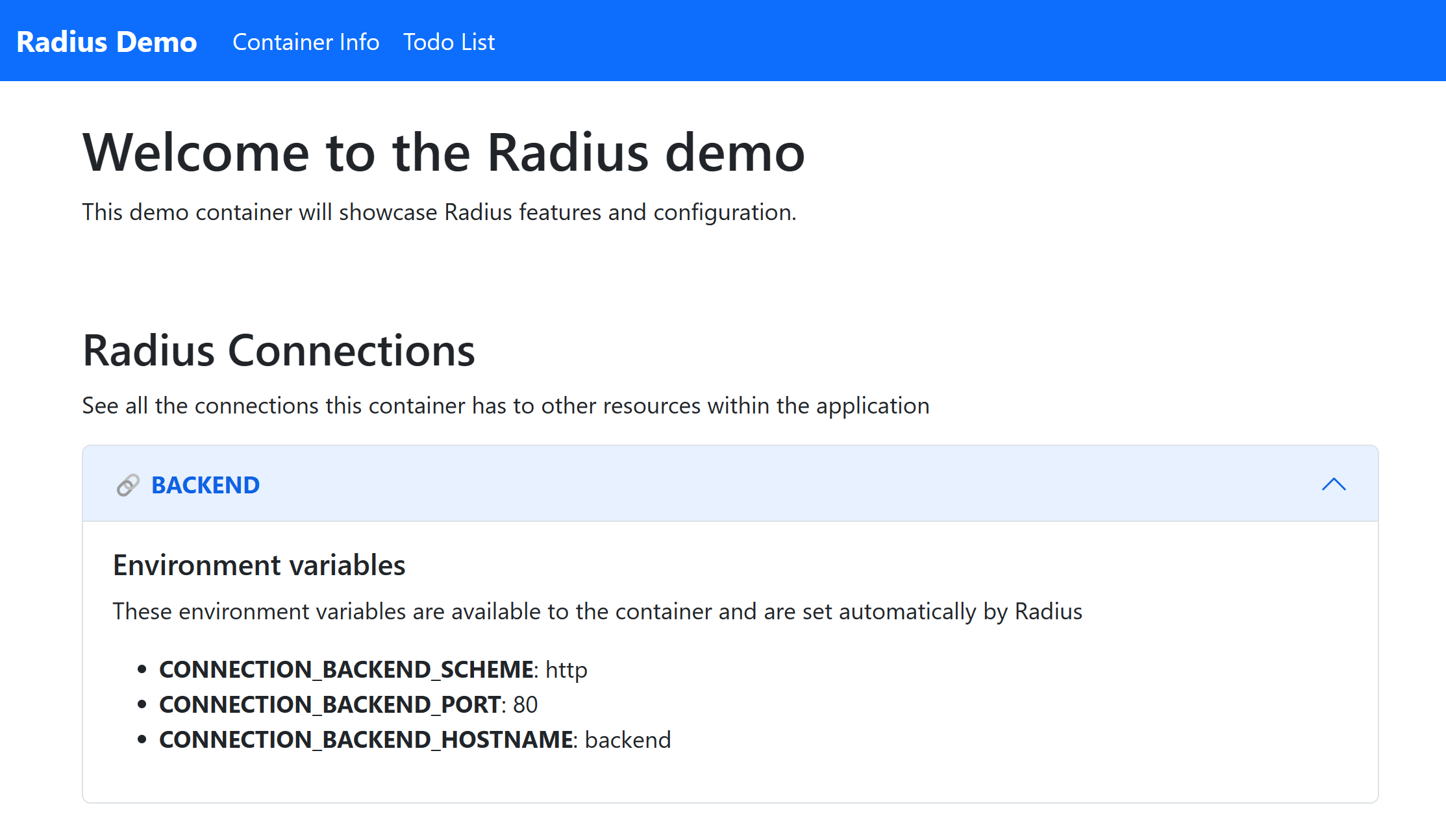
Done
You have successfully added a connection between two containers. Make sure to delete your application to clean up the containers:
rad app delete networking-demo -y
Feedback
Was this page helpful?
Glad to hear it! Please feel free to star our repo and join our Discord server to stay up to date with the project.
Sorry to hear that. If you would like to also contribute a suggestion visit and tell us how we can improve.