Tutorial: Dapr Microservices
Categories:
This tutorial will teach you the following about Dapr:
- How to use Radius to deploy a Dapr microservices sample application for an online shop
- How Dapr and Radius seamlessly work together
For more details on the app and access to the source code, visit the samples/dapr
directory in the samples repo.
Prerequisites
- rad CLI
- Bicep VSCode extension
- Radius environment
- Setup a supported Kubernetes cluster
- Dapr installed on your Kubernetes cluster
Step 1: Initialize a Radius Environment
-
Begin in a new directory for your application:
mkdir dapr cd dapr
-
Initialize a new dev environment:
Select ‘Yes’ when prompted to create an application.
rad init
Step 2: Define the application, backend
container, and Dapr state store
Begin by creating a new file named dapr.bicep
with a Radius Application that consists of a backend
container and Dapr state store with Redis:
extension radius
@description('Specifies the environment for resources.')
param environment string
@description('The ID of your Radius Application. Automatically injected by the rad CLI.')
param application string
// The backend container that is connected to the Dapr state store
resource backend 'Applications.Core/containers@2023-10-01-preview' = {
name: 'backend'
properties: {
application: application
container: {
// This image is where the app's backend code lives
image: 'ghcr.io/radius-project/samples/dapr-backend:latest'
ports: {
orders: {
containerPort: 3000
}
}
}
connections: {
orders: {
source: stateStore.id
}
}
extensions: [
{
kind: 'daprSidecar'
appId: 'backend'
appPort: 3000
}
]
}
}
// The Dapr state store that is connected to the backend container
resource stateStore 'Applications.Dapr/stateStores@2023-10-01-preview' = {
name: 'statestore'
properties: {
// Provision Redis Dapr state store automatically via the default Radius Recipe
environment: environment
application: application
}
}
Step 3: Deploy the backend
application
-
Deploy the application’s
backend
container and Dapr state store:rad run dapr.bicep
-
You can confirm all the resources were deployed by looking for
dapr
,backend
, andstatestore
resources in the console logs:Deployment Complete Resources: dapr Applications.Core/applications backend Applications.Core/containers statestore Applications.Dapr/stateStores
-
The
rad run
command automatically sets up port forwarding. Visit the the URL http://localhost:3000/order in your browser. You should see the following message, which confirms the container is able to communicate with the state store:{"message":"no orders yet"}
-
Press CTRL+C to terminate the port-forward.
-
A local-dev Recipe was run during application deployment to automatically create a lightweight Redis container plus a Dapr component configuration. Confirm that the Dapr Redis statestore was successfully created:
dapr components -k -A
You should see the following output:
NAMESPACE NAME TYPE VERSION SCOPES CREATED AGE default-dapr statestore state.redis v1 2023-07-21 16:04.27 21m
Step 4: Define the frontend
container
Add a frontend
container which will serve as the application’s user interface.
// The frontend container that serves the application UI
resource frontend 'Applications.Core/containers@2023-10-01-preview' = {
name: 'frontend'
properties: {
application: application
container: {
// This image is where the app's frontend code lives
image: 'ghcr.io/radius-project/samples/dapr-frontend:latest'
env: {
// An environment variable to tell the frontend container where to find the backend
CONNECTION_BACKEND_APPID: {
value: 'backend'
}
// An environment variable to override the default port that .NET Core listens on
ASPNETCORE_URLS: {
value: 'http://*:8080'
}
}
// The frontend container exposes port 8080, which is used to serve the UI
ports: {
ui: {
containerPort: 8080
}
}
}
// The extension to configure Dapr on the container, which is used to invoke the backend
extensions: [
{
kind: 'daprSidecar'
appId: 'frontend'
}
]
}
}
Step 5. Deploy and run the frontend
application
-
Use Radius to deploy and run the application with a single command:
rad run dapr.bicep
-
Your console should output a series of deployment logs, which you may check to confirm the
frontend
container was successfully deployed:Deployment Complete Resources: dapr Applications.Core/applications backend Applications.Core/containers frontend Applications.Core/containers statestore Applications.Dapr/stateStores
Step 6. Test your application
In your browser, navigate to the endpoint (e.g. http://localhost:8080) to view and interact with your application:
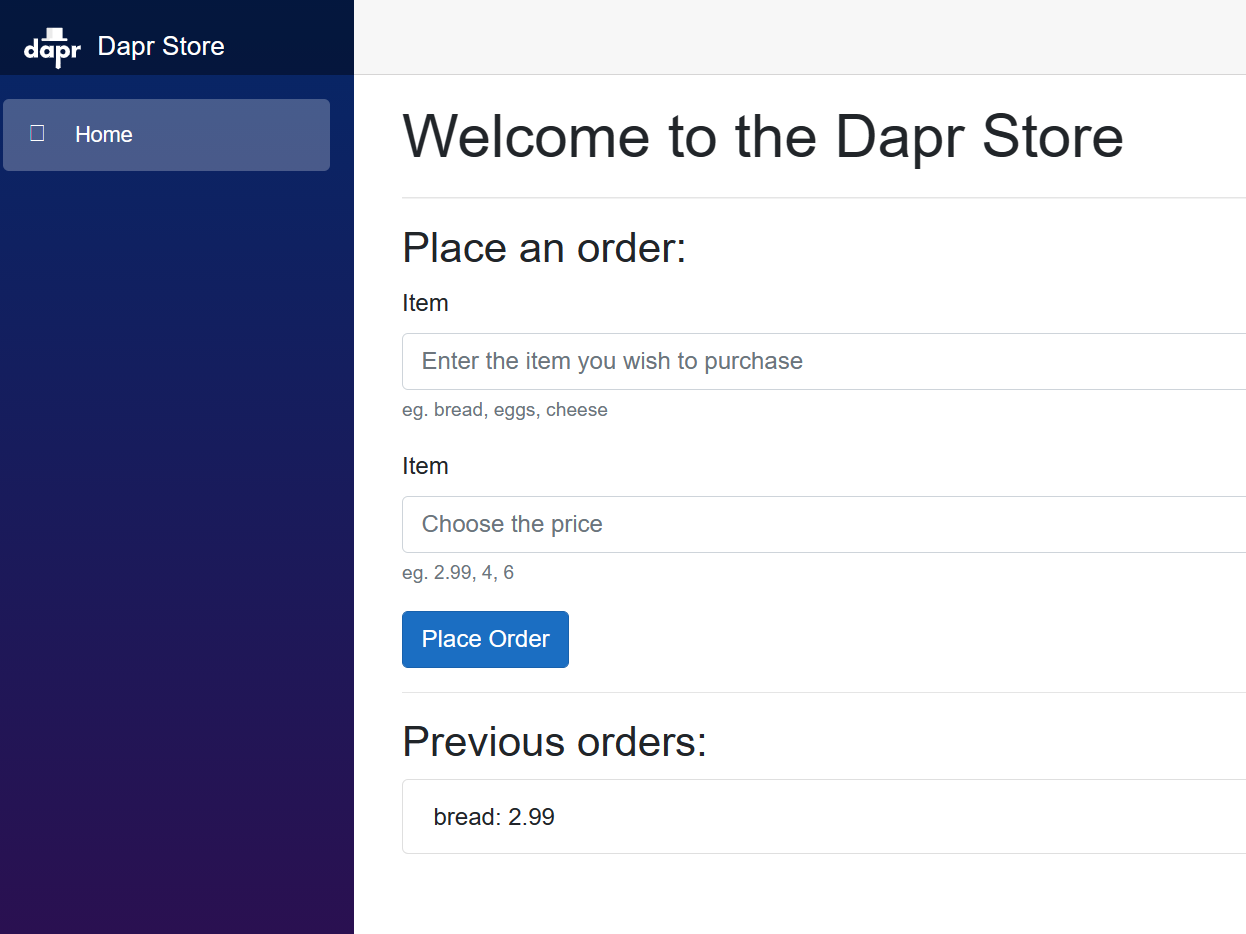
Cleanup
-
Press
CTRL
+C
to terminate the log console -
Run the following command to cleanup your Radius Application, containers, and Dapr statestore. The Recipe resources (Redis container and Dapr component) are also automatically cleaned up.
rad app delete
Next steps
- Related links for Dapr:
- If you’d like to try another tutorial with your existing environment, go back to the Radius tutorials page.
Feedback
Was this page helpful?
Glad to hear it! Please feel free to star our repo and join our Discord server to stay up to date with the project.
Sorry to hear that. If you would like to also contribute a suggestion visit and tell us how we can improve.